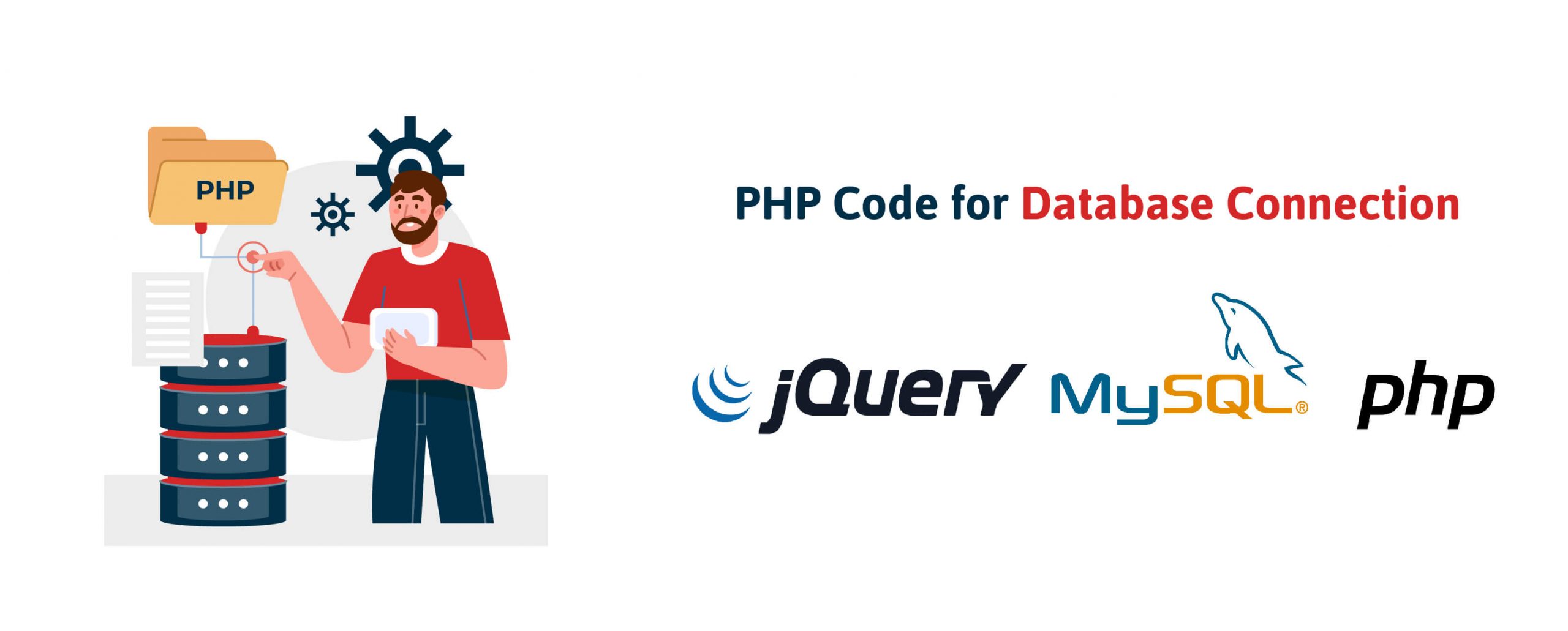
Prerequisites:
In order to complete this tutorial, you have to have a system (XAMP or LAMP or MAMP) having PHP and MySQL installed.
Step 1: PHP Code For Database Connection in Database Connection File.
First of all, we will create a new PHP file and will name it database_connection.php. Why are we creating a separate database connection file? Because in case we need database connection in multiple files in a project in which we have to perform CRUD tasks in the databases, we don’t have to write the database connection code every time. Hence, we are creating 2 functions, one to create a database connection and another one to close the connection as below:
<?php //StartDatabaseConnection() function returns connection string. function StartDatabaseConnection() { $database_host = "localhost"; //database host name $database_user = "root"; // database user name $database_password = "root"; // database user password $database_name = "example"; // database name $connection = new mysqli($database_host, $database_user, $database_password,$database_name) or die("Connection has failed: %s\n". $connection -> error()); return $connection; } //CloseDatabaseConnection() function returns true if connections gets closed successfully and false if connection doesn't get closed. We have to pass array variable which carries the connection related data. function CloseDatabaseConnection($connection) { return $connection -> close(); } ?>
Step 2: Create a file index.php that will use functions created in the above file.
<?php //First of all, we'll have to include the database_connection.php file so that we can use the functions which we have defined in that file. include 'database_connection.php'; // We'll connect with the database using StartDatabaseConnection() function. We are getting the result of calling this function in connectdb variable. If the connection to database becomes successful, this variable value will be true else it will be false. $connectdb = StartDatabaseConnection(); if ($connectdb == true){echo "Database has been successfully connected.";} else {echo "Database connection failed.";} // We'll close the database connection now using CloseDatabaseConnection() function. We are getting the result of calling this function in closingresult variable. If the connection closed successfully, this variable value will be true else it will be false. $closingresult = CloseDatabaseConnection($connectdb); if ($closingresult == true) {echo "<br />". "Database connection has been successfully closed.";} else {echo "Database connection has not been closed.";} ?>
Hope you like this PHP Code For Database Connection. If you’ve any questions or concerns or issues, please feel free to mention the same in the comments section below. Click here for more quick PHP Snippets.
Click the below link to check the demo. Download link to download the files used in this tutorial is also available on this page.